作るゲームらしきもの「Save Strawberry!」
この記事は、ゲーム「Save Strawberry!」の作成手順を解説するページではありません。
管理人としては、ゲームを作る楽しさを知ってほしい為、「Save Strawberry!」で使ったアセット素材、BGM素材、Unityの知識や操作、ゲーム中のスクリプト、設定のヒントを紹介するにとどめます。
Let’s try!
使用したアセット(全て無料)
- Standard Assets (for Unity 2018.4)(プレイヤー)
- Low Poly Fruit Pickups(イチゴ)
- Fx Explosion Pack(爆破エフェクト)
- Skybox Series Free(空と地面のマテリアル)
なお、上記で紹介したStandard Assets(for Unity 2018.4)とFx Explosion Packの2つのアセットはUnity 2019以降だとインポートしただけでエラーが出ます。対処方法は以下をご覧ください。
ゲーム中の音楽
- 魔王魂 サイバー41(BGM)
- 魔王魂 爆発05(SE)
必要なUnityの知識
その他動画資料
ゲームで使用したスクリプト
使っているスクリプトは以下の2つです。
FruitsController
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FruitController : MonoBehaviour
{
public GameObject Effect; // 爆破エフェクト用プレハブ
public AudioClip Se; // 爆破音源ファイル
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
// 当たり判定
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.CompareTag("Cube")) // Tag「Cube」と接触したら
{
Instantiate(Effect, transform.position, Quaternion.identity); // 爆破エフェクト
AudioSource.PlayClipAtPoint(Se, transform.position); // 爆破音
Destroy(gameObject); // イチゴ消去
Destroy(collision.gameObject); // Cube消去
}
}
}
- シーンに配置したイチゴにアタッチする
- イチゴにアタッチ後は、Fruits ControllerのEffectとSeにExplosion6(アセットFx Explosion Packに収録)と魔王魂 爆発05(魔王魂サイトからダウンロードしたフリー音源)をそれぞれ設定する
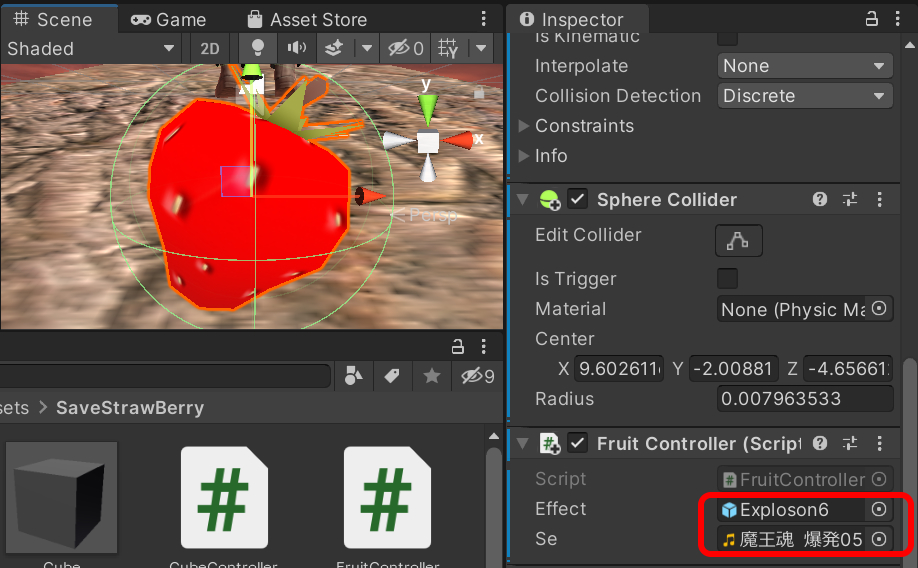
CubeController
- Create Emptyで作った空のオブジェクトをGameMainに名前変更して本スクリプトをアタッチする
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CubeController : MonoBehaviour
{
public GameObject Prefab; // Cubeプレハブ
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
// 60フレーム毎にCubeを生成させる
if(Time.frameCount % 60 == 0){
float x = Random.Range(-5.0f, 5.0f); // 生成範囲(x軸方向)
Vector3 pos = new Vector3(x, 3.0f, 4.0f); // 生成位置
Instantiate(Prefab, pos, Quaternion.identity); // プレハブを生成
}
}
}
GameMainにアタッチ後は、後述のCubeプレハブをCube ControllerのPrefabに設定しておくとランダムでCubeが出現するようになる
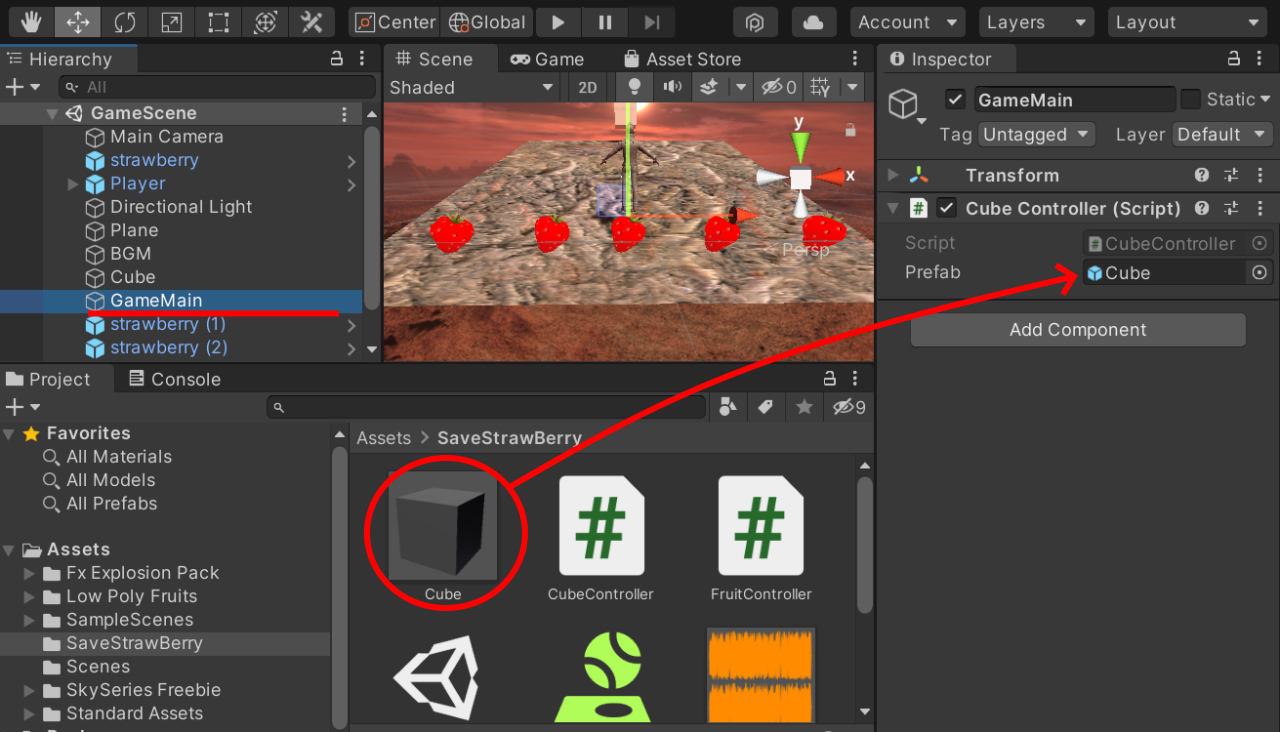
作る上でのヒント
シーン視点は、Y軸に対してX軸が右になるように作っています。
Planeを配置したら、まず原点(TransformのPosition項目XYZを0)にすると楽です。
Unity Editor全体イメージ
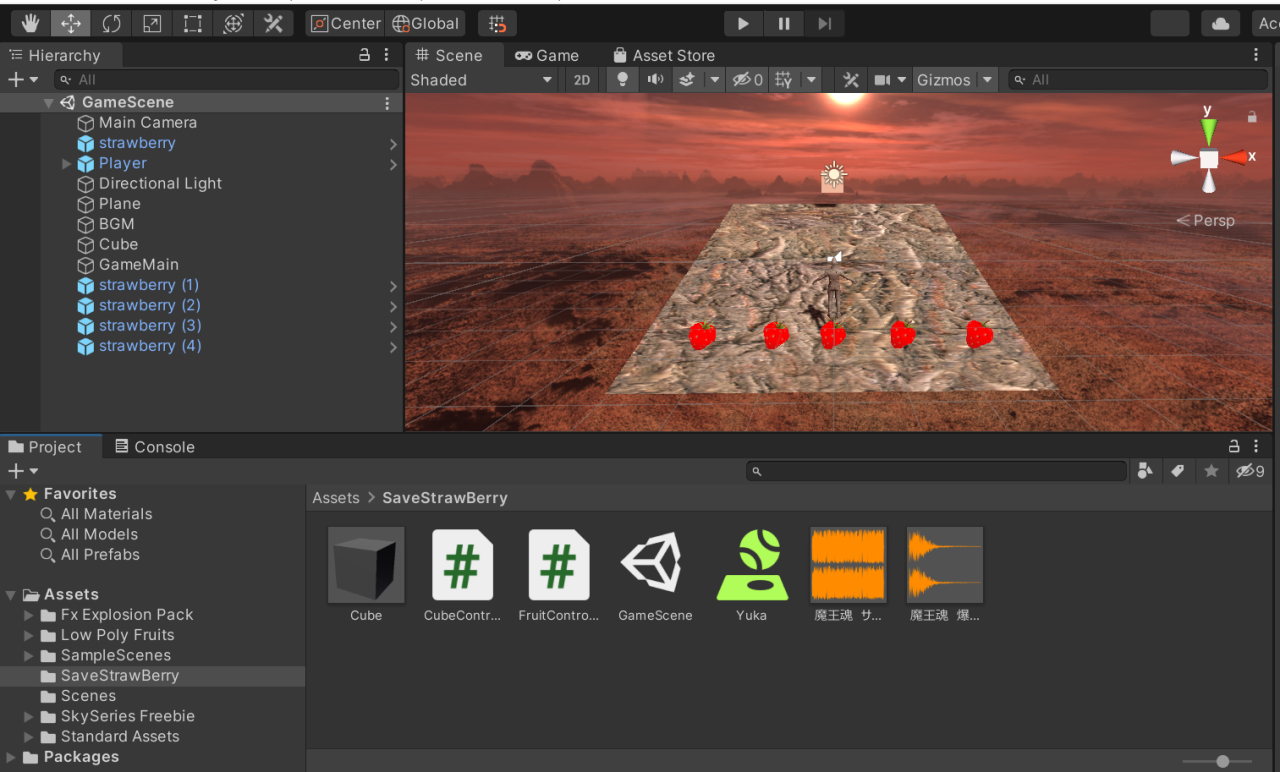
プレイヤー
- Standard AssetsのThirdPersonCharacterを使用し、名前をPlayerに変更
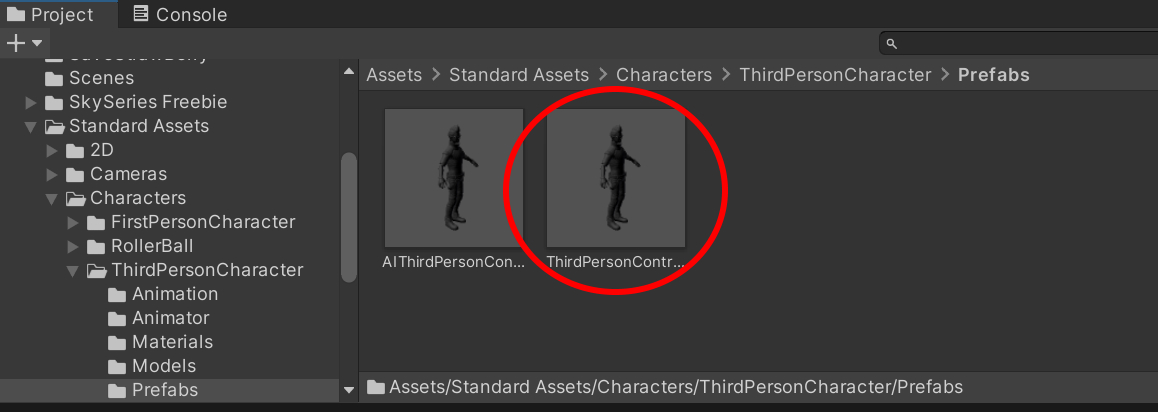
イチゴ
- Rigidbody, Sphere Collider, Fruits Controller(スクリプト)を追加
- RigidbodyのFreeze Position X, Zにチェック
- Fruits ControllerのEffect, SeにそれぞれExploson6、魔王魂 爆発05を設定
- 上記3点が完了したら、5個コピーして適当にシーン上に配置
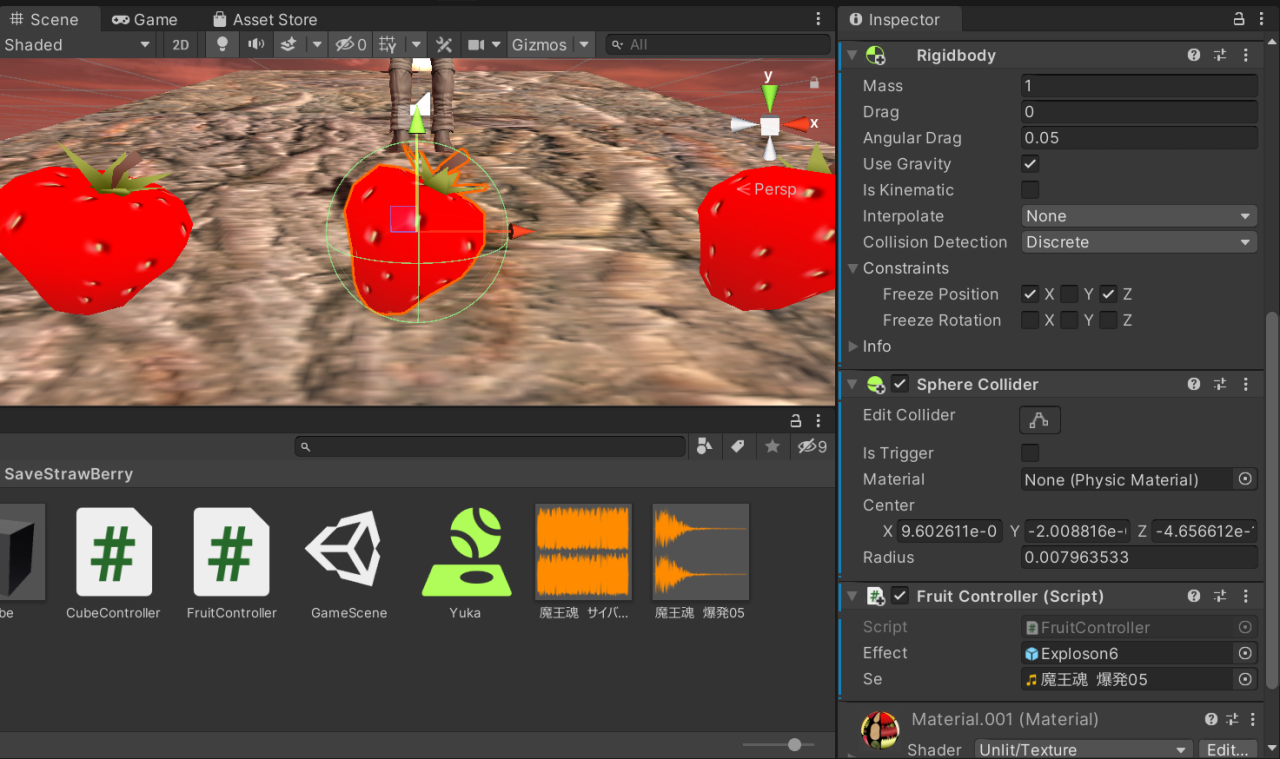
落ちてくるCube
- 3D ObjectのCubeを使用
- Rigidbodyを追加
- タグ名をCubeとして設定しプレハブ化
- プレハブ化したらシーン上のCubeは消去
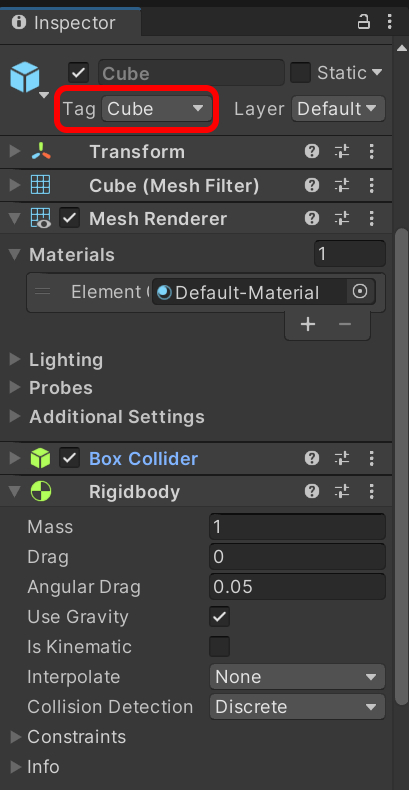
床(Plane)
- 画面奥から手前に向かって下り落ちる様に傾けた(RotationのX軸 サンプルでは-20)
- 物理マテリアルYuka(後述)を作成し、アタッチする
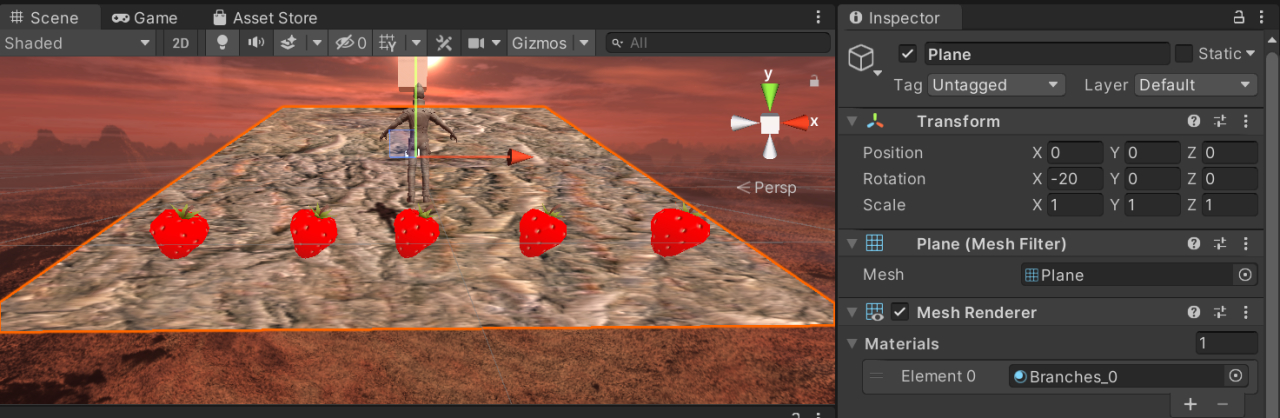
滑る床(Yuka)
- Physics Materialをアセットに作成しYukaに名前変更
- Friction関係を0にして、滑る感じの設定(下図参照)にした
- 作成後は、シーン上のPlaneにアタッチする
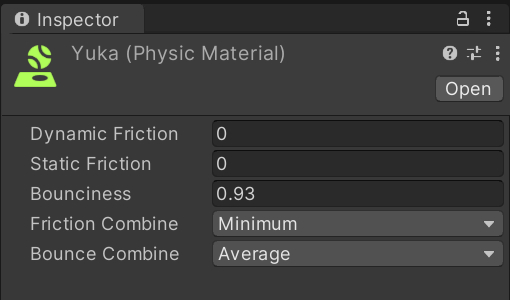
BGM
- Audio Sourceをシーンに追加しBGMに名前変更
- BGMのAudio Clipに魔王魂 サイバー41を設定、Play On AwakeとLoopにチェック
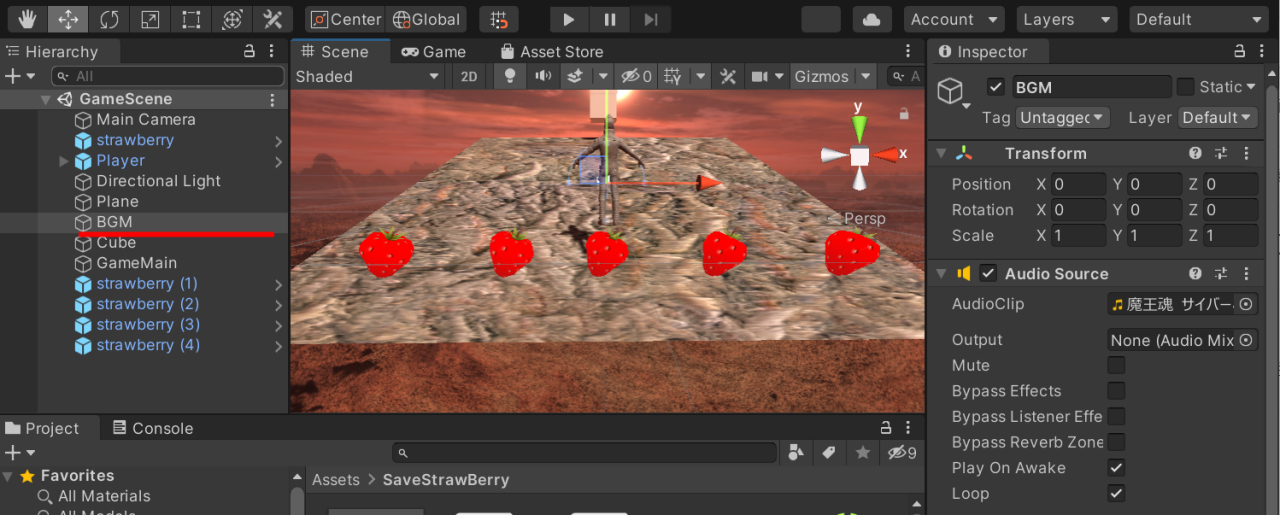
作成後は、ビルドしてみるとよりゲームっぽくなります。
参考
コメント