「JavaScriptのオブジェクト形式を日常の事物に利用したらどうなるか?」を考えてみます。
いくつかのサンプルからオブジェクト形式の利用方法が見えてくると思います。
画面上のXY座標をオブジェクトで表す
実行イメージ(コンソールに表示/Chromeの場合F12)
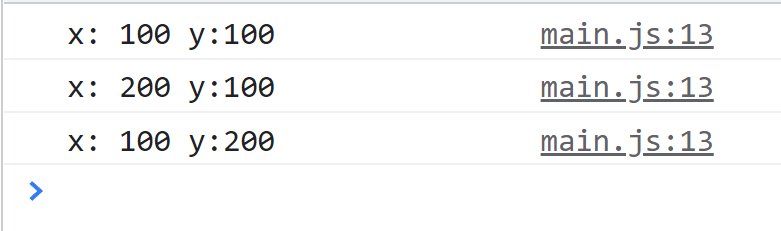
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="main.js"></script>
<title>XY座標をオブジェクトで表す</title>
</head>
<body>
<p>コンソールに表示しています...</p>
</body>
</html>
main.js
// main.js
window.addEventListener("load", ()=>{
// xy座標を表すオブジェクトを格納する配列
let pos = [];
// xy座標のプロパティを持つオブジェクトを配列に代入
pos.push( { x: 100, y: 100 } );
pos.push( { x: 200, y: 100 } );
pos.push( { x: 100, y: 200 } );
// 配列posの内容を表示
for(let p of pos){
console.log(`x: ${p.x} y:${p.y}`);
}
});
XY座標オブジェクトをCanvasに利用してみる
実行イメージ
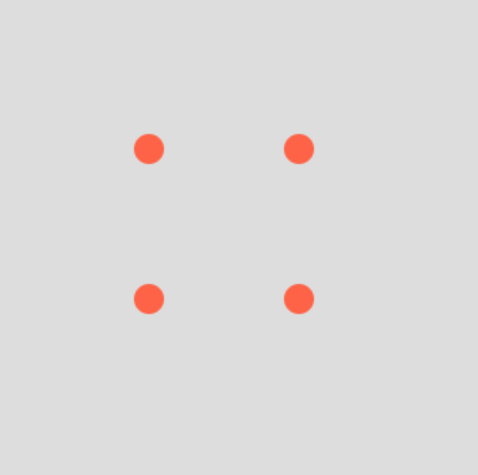
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
*{ margin: 0; padding: 0; }
</style>
<script src="main.js"></script>
<title>XY座標オブジェクトをCanvasに利用してみる</title>
</head>
<body>
<canvas id="canvas" width="320" height="320"></canvas>
</body>
</html>
main.js
// main.js
window.addEventListener("load", ()=>{
// xy座標を表すオブジェクトを格納する配列
let pos = [];
// xy座標のプロパティを持つオブジェクトを配列に代入
pos.push( { x: 100, y: 100 } );
pos.push( { x: 200, y: 100 } );
pos.push( { x: 100, y: 200 } );
pos.push( { x: 200, y: 200 } );
// キャンバス取得
const canvas = document.getElementById("canvas");
const g = canvas.getContext("2d");
// 背景塗りつぶし
g.fillStyle = "#ddd";
g.fillRect(0, 0, canvas.width, canvas.height);
// posで指定した座標に円を描く
g.fillStyle = "tomato";
for(let p of pos){
g.beginPath();
g.arc(p.x, p.y, 10, 0, Math.PI*2, true);
g.fill();
}
});
RGBカラーをオブジェクトで表す
実行イメージ(コンソールに表示/Chromeの場合F12)
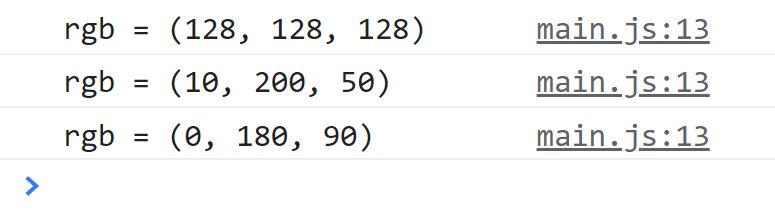
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="main.js"></script>
<title>RGBカラーをオブジェクトで表す</title>
</head>
<body>
<p>コンソールに表示しています...</p>
</body>
</html>
main.js
// main.js
window.addEventListener("load", ()=>{
// RGBカラーを表すオブジェクトを格納する配列
let colors = [];
// RGBカラー値(0~255)を持つオブジェクトを配列に代入
colors.push( { r: 128, g: 128, b: 128 } );
colors.push( { r: 10, g: 200, b: 50 } );
colors.push( { r: 0, g: 180, b: 90 } );
// 配列colorsの内容を表示
for(let color of colors){
console.log(`rgb = (${color.r}, ${color.g}, ${color.b})`);
}
});
RGBカラーオブジェクトをCanvasに利用してみる
実行イメージ
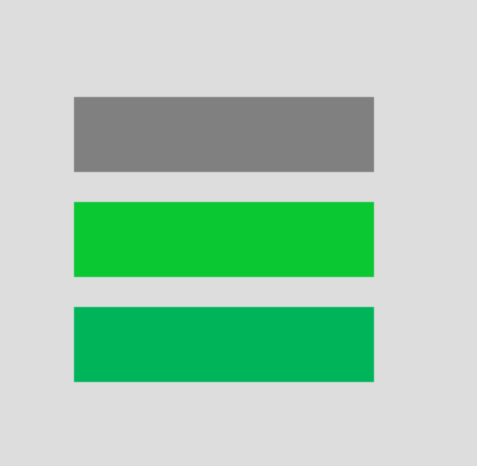
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
*{ margin: 0; padding: 0; }
</style>
<script src="main.js"></script>
<title>RGBカラーオブジェクトをCanvasに利用してみる</title>
</head>
<body>
<canvas id="canvas" width="320" height="320"></canvas>
</body>
</html>
main.js
// main.js
window.addEventListener("load", ()=>{
// RGBカラーを表すオブジェクトを格納する配列
let colors = [];
// RGBカラー値(0~255)を持つオブジェクトを配列に代入
colors.push( { r: 128, g: 128, b: 128 } );
colors.push( { r: 10, g: 200, b: 50 } );
colors.push( { r: 0, g: 180, b: 90 } );
// キャンバス取得
const canvas = document.getElementById("canvas");
const g = canvas.getContext("2d");
// 背景を塗りつぶす
g.fillStyle = "#ddd";
g.fillRect(0, 0, canvas.width, canvas.height);
// colorsに格納した色で矩形を描画
let y = 0;
for(let color of colors){
g.fillStyle = `rgb(${color.r}, ${color.g}, ${color.b})`; // rgb(xxx, xxx, xxx)
g.fillRect(50, y+=70, 200, 50);
}
});
人をオブジェクトで表してみる
実行イメージ(コンソールに表示/Chromeの場合F12)
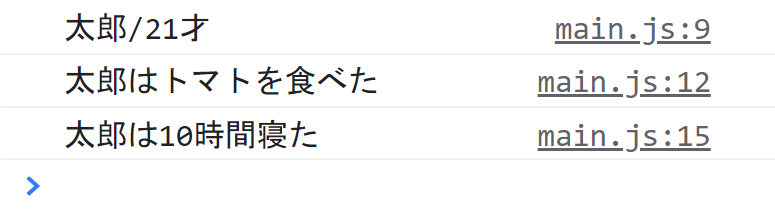
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="main.js"></script>
<title>人をオブジェクトで表してみる</title>
</head>
<body>
<p>コンソールに表示しています...</p>
</body>
</html>
main.js
// main.js
window.addEventListener("load", ()=>{
// 太郎を定義
const taro = {
name: "太郎",
age: 21,
info: function(){
console.log(this.name + "/" + this.age + "才");
},
eat: function(food){
console.log(this.name + "は" + food + "を食べた");
},
sleep: function(long){
console.log(this.name + "は" + long + "時間寝た");
}
};
// オブジェクトtaroのメソッド利用
taro.info();
taro.eat("トマト");
taro.sleep(10);
});
その他、ポケモンのキャラクタ、日時、書籍情報などなど…
オブジェクトで表すと面白いものが沢山あると思います。
コメント