classとCanvasAPIを組み合わせて、キャンバスに円を描画する利用例を紹介します。
Arcクラス
円の情報(x座標、y座標、半径)とキャンバス描画部分を1つのクラス(Arc)にまとめました。
Arc.js
/* Arc.js : キャンバスの指定した位置に円を描くクラス*/
class Arc{
// コンストラクタ(x座標, y座標, 半径)
constructor(x, y, r){
this.x = x; // x座標
this.y = y; // y座標
this.r = r; // 半径
console.log("x: " + this.x + " y: " + this.y + " r=" + this.r);
}
// 描画メソッド
draw(){
g.beginPath();
g.arc(this.x, this.y, this.r, 0, Math.PI*2, false);
g.stroke();
}
}
仕様
コンストラクタ | 円のx座標、y座標、半径を設定 |
drawメソッド | コンストラクタで指定したx座標、y座標、半径を用いて円を描画 |
補足
クラス内で利用しているgはCanvasAPIのgetContext(“2d”)で取得されたグローバル変数です。
そのためArcクラス単体では利用できません。
利用例
let arc = new Arc(250, 250, 100); arc.draw();
Arcクラスを利用してキャンバスに円を描画する
実行イメージ(500×500ピクセルのキャンバスの中央に円が描画されます)
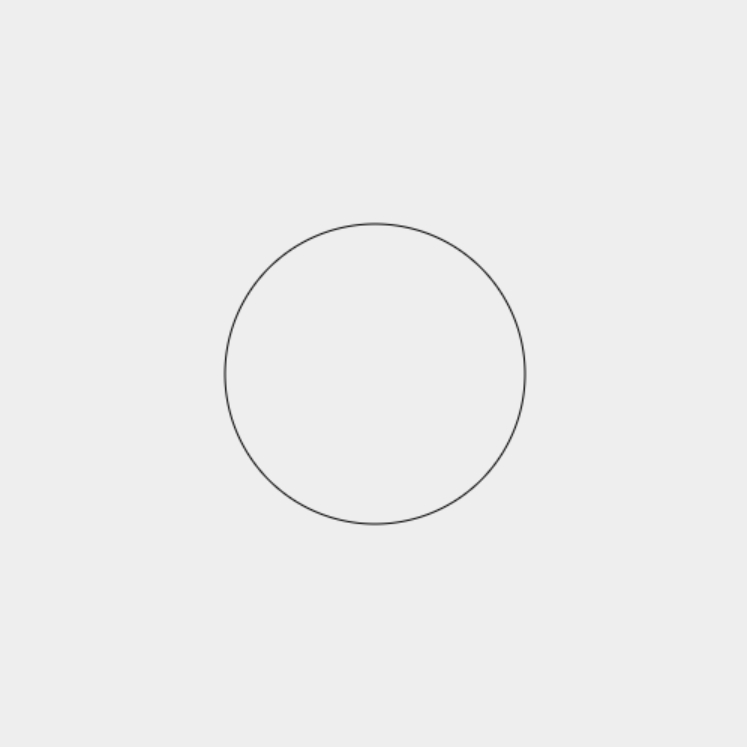
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<script src="Arc.js" type="text/javascript"></script>
<script src="main.js" type="text/javascript"></script>
<title>Arcクラス(円を1つ描画)</title>
</head>
<body>
<canvas id="canvas" width="500" height="500"></canvas>
</body>
</html>
style.css
/* style.css */
@charset "utf-8";
*{
margin: 0;
padding: 0;
}
canvas{
background-color: #eee;
}
main.js
// main.js
let canvas = null;
let g = null;
window.addEventListener("load", ()=>{
// キャンバス取得
canvas = document.getElementById("canvas");
g = canvas.getContext("2d");
// クラスArcを使って円を描く
let arc = new Arc(250, 250, 100);
arc.draw();
});
複数の円を描画する
円をたくさん描画したいときはどうするのか?
クラスArcをnewしたインスタンス変数を再利用しないのであれば、次のようにfor文で簡単に処理できます。
円を並べて表示させる
for文の入れ子を使って10×10個(=100個)の円を並べて描画します。
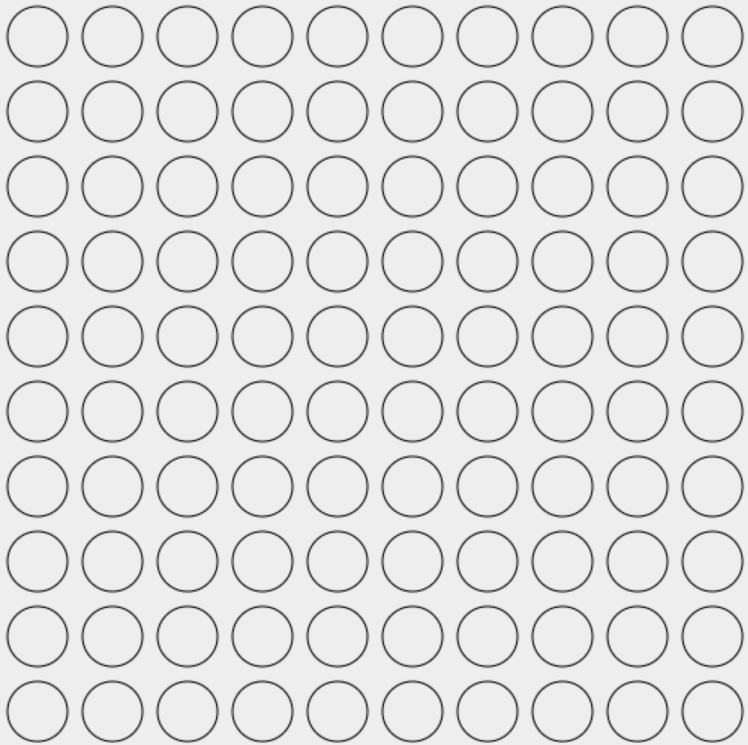
index.html, style.css, Arc.js の基本構成は同じため省略
main.js
// main.js
let canvas = null;
let g = null;
window.addEventListener("load", ()=>{
// キャンバス取得
canvas = document.getElementById("canvas");
g = canvas.getContext("2d");
// クラスArcを使って指定位置に円を描く(100個)
for(let i=0; i<10; i++){
for(let j=0; j<10; j++){
let arc = new Arc(i*50+25, j*50+25, 20);
arc.draw();
}
}
});
複数の円をランダムで描画する
ランダムな位置と半径の円を100個描画してみました。
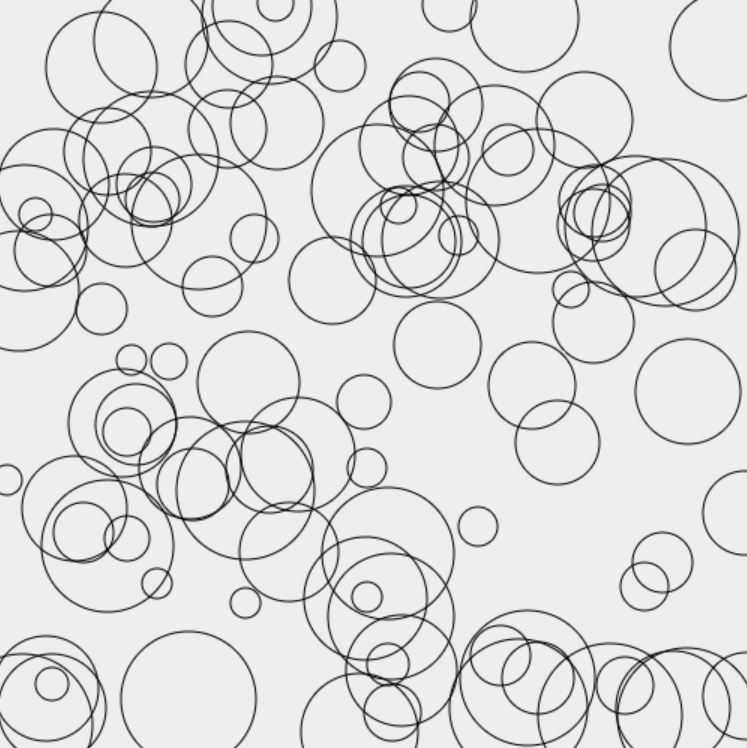
index.html, style.css, Arc.js の基本構成は同じため省略
main.js
// main.js
let canvas = null;
let g = null;
window.addEventListener("load", ()=>{
// キャンバス取得
canvas = document.getElementById("canvas");
g = canvas.getContext("2d");
// クラスArcを使ってランダムな位置と半径で円を描く(100個)
for(let i=0; i<100; i++){
// xy座標
const x = Math.floor(Math.random() * canvas.width);
const y = Math.floor(Math.random() * canvas.height);
// 半径
const r = Math.floor(Math.random() * 40) + 10;
// 円を描画
let arc = new Arc(x, y, r);
arc.draw();
}
});
以上、Arcクラスを作ってキャンバスに円を描画するでした。
コメント